The Ultimate Guide to Converting Milliseconds to Date
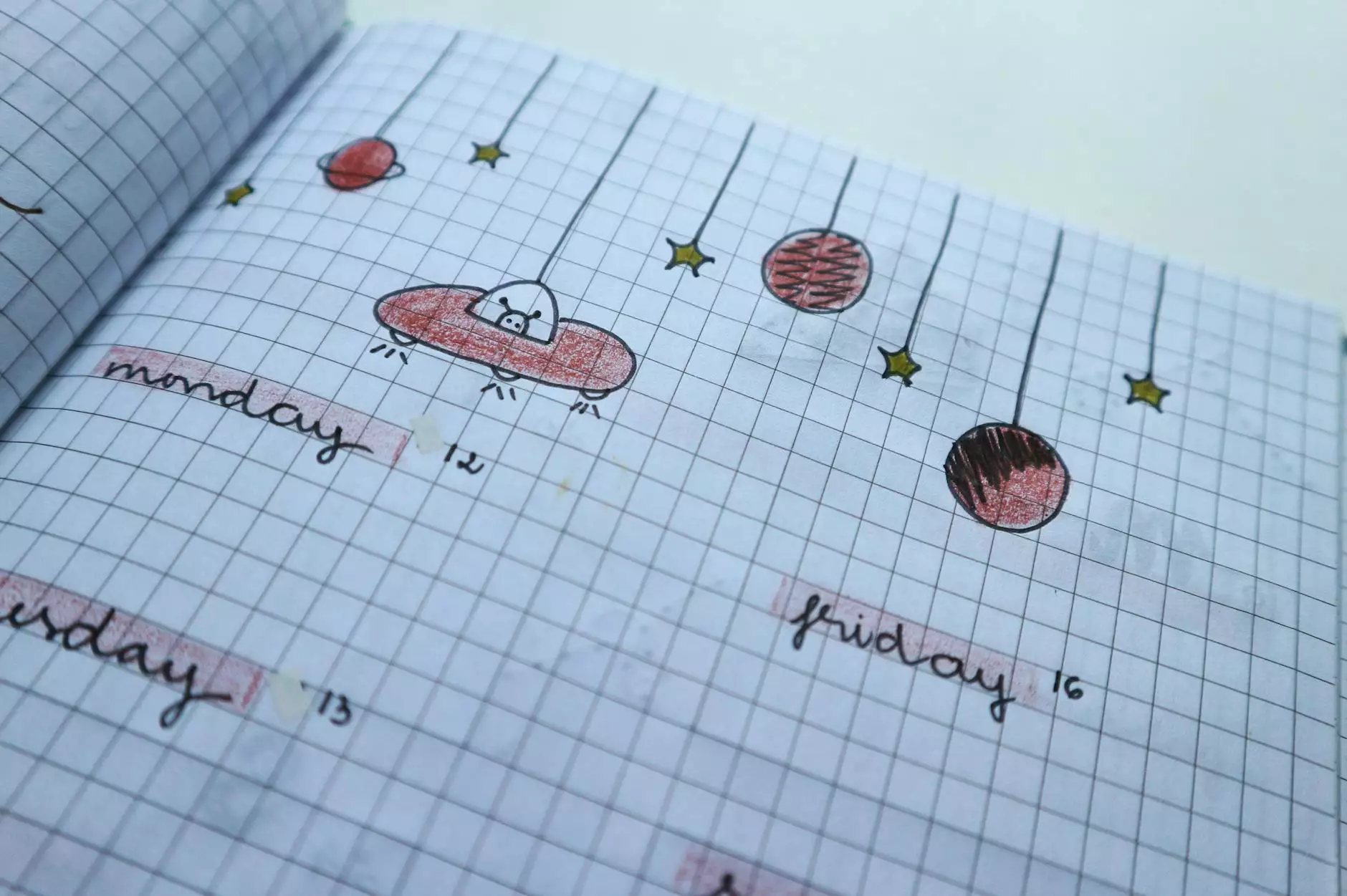
When working with programming languages, converting milliseconds to a date is a common requirement in various scenarios. Understanding the process and syntax for this conversion can streamline your development tasks and enhance the functionality of your applications.
Why Convert Milliseconds to Date?
Converting milliseconds to a date allows you to display time-related data in a user-friendly format. Whether you are working on web design projects or software development tasks, accurately converting milliseconds to a date is crucial for presenting time-sensitive information effectively.
Methods for Converting Milliseconds to Date
Depending on the programming language you are using, there are different methods and functions available for converting milliseconds to a date. Let's explore some commonly used languages and their syntax for this conversion:
JavaScript
In JavaScript, you can use the Date object to convert milliseconds to a date. Here's an example code snippet:
const date = new Date(milliseconds); console.log(date);Python
Python provides the datetime module for handling date and time operations. Here's how you can convert milliseconds to a date in Python:
import datetime date = datetime.datetime.fromtimestamp(milliseconds / 1000.0) print(date)Java
For Java developers, the SimpleDateFormat class can be used to convert milliseconds to a date. Here's a sample code snippet in Java:
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Date date = new Date(milliseconds); String formattedDate = sdf.format(date); System.out.println(formattedDate);Integrating the Conversion in Web Design and Software Development
Whether you are creating a dynamic web application or a software solution, the ability to convert milliseconds to a date seamlessly can enhance the user experience and functionality of your product. By implementing the appropriate conversion logic in your code, you can display time-related information accurately and intuitively.
Conclusion
Converting milliseconds to a date is a fundamental aspect of programming that is essential for handling time-related data effectively. By understanding the methods and syntax for this conversion in various programming languages, you can enrich your development projects and deliver enhanced user experiences.